Conan Mercer Site Reliability Engineer
A Guide to Creating Your Very First Docker Application
02 Aug 2024 - Conan Mercer
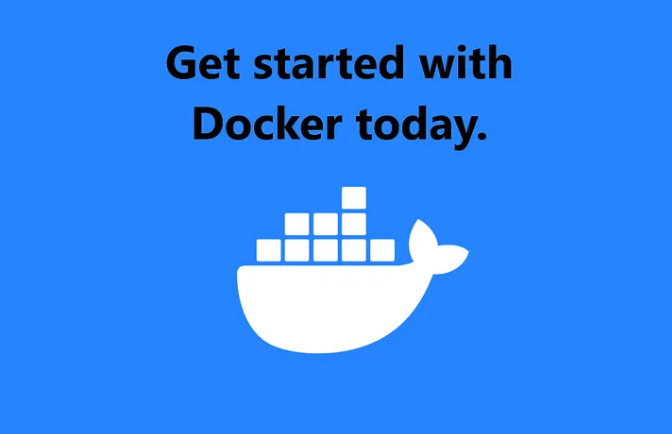
How Docker Works
Docker is used to develop, test, ship, and run applications. The key aspect of Docker is that it allows the programmer to separate the application from the infrastructure it runs on. Before Docker, Virtual Machines (VMs) were commonplace. The difference between Docker and a traditional VM is that Docker runs on a Docker Engine. It does not require a Hypervisor and a guest OS. This makes the Docker container lighter and increases boot time. A Docker container can also run in pretty much any environment, making it more portable than a VM. Containers can be built and destroyed much faster than a VM. It is much easier to deploy a Docker container on a cloud server than it is for a VM.
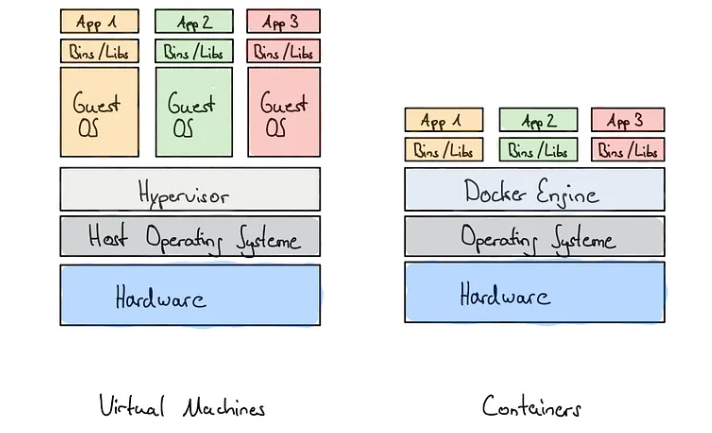
Creating your first Docker application
First things first, installing Docker on Linux
Update packages, install docker, and test docker installation:
sudo apt update
sudo apt install docker.io
sudo docker run hello-world
Write the project
We are going to need two files to run our application. A Dockerfile and a Python file.- The Python file main.py contains the code that will be run by the Docker container
- The Dockerfile is used to configure how the Docker container will run
#!/usr/bin/env python3
print("Hello from Docker!")
The Dockerfile is where the magic happens:
FROM python:latest
COPY main.py /
CMD [ "python", "./main.py" ]
The goal of the Dockerfile is to launch the Python code.
First, a Python image is loaded using the FROM instruction.
Then the Python file, in this case, main.py is copied into the container using the COPY instruction.
Lastly, the CMD instruction tells the container to execute the Python file.
The code used in this guide can be found on my GitHub here
Create the Docker Image for the first time
Using the terminal, the image first needs to be created before it can be run. The following command creates the image called “docker-python-test”
docker build -t docker-python-test .
The -t command allows us to name the image, in this case, docker-python-test
Run the Docker Image for the first time
Now that we have created an image to run, it is time actually run it.
docker run docker-python-test
Hurray!
You should see “Hello from Docker!” output on your terminal screen.
Conclusion
From here you are ready to start building and running applications in Docker! The skies are the limit!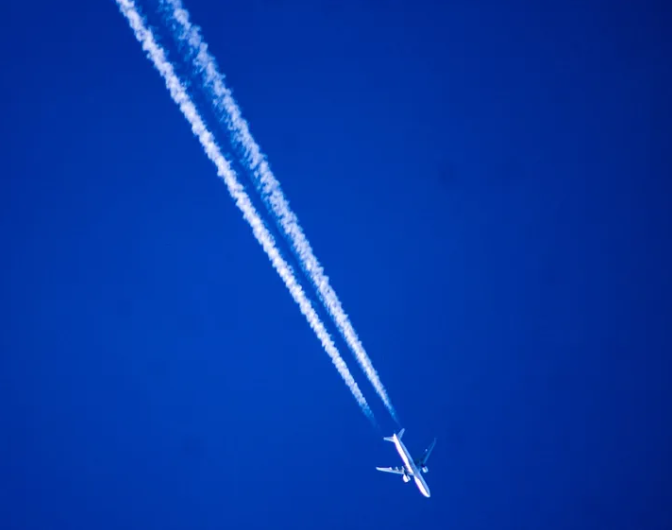